이번 포스팅은 developer 사이트를 참고하여 제작되었습니다.
developer.android.com/training/notify-user/group
알림 그룹 만들기 | Android 개발자 | Android Developers
Android 7.0(API 수준 24)부터는 관련된 알림을 그룹(이전에는 '번들된' 알림이라고 함)으로 표시할 수 있습니다. 예를 들어, 앱에서 수신된 이메일의 알림을 표시하려면 모든 알림을 동일한 그룹에 ��
developer.android.com
Android 7.0 (Marshmallow, API24)부터 추가된 알림 그룹으로 여러 개의 알림을 한 개의 알림으로 묶어 보여줄 때 사용합니다.
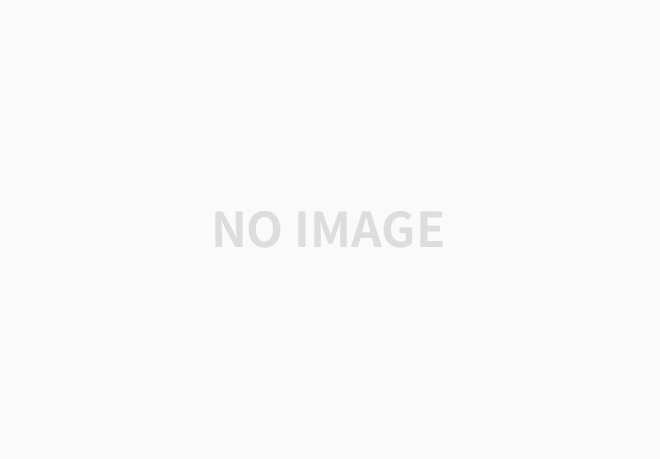
이때 우측 상단의 펼치기 버튼을 클릭하면 아래와 같이 펼쳐져 보입니다.
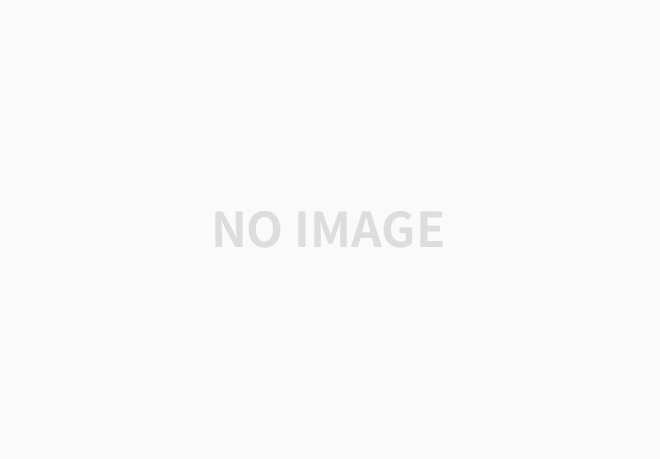
※ 참고
- Summary Notification을 만들지 않으면 그룹핑 하지 않은 알람처럼 개별적으로 표시됩니다.
물론 Summary Notification을 만들지 않으면 각각의 Notification이 개별적으로 보여서 더 많은 정보를 보여줄 수 있지만, 이로 인해 사용자에게는 애플리케이션에 대한 안 좋은 경험을 쌓게 하여 어플을 삭제하거나 알람을 꺼버리는 경우가 발생하여 더 최악의 상황이 발생할 수 있기 때문에 고려하여 이용하여야 합니다.
Step 01. 그룹핑 할 그룹명을 지정해줍니다.
String notiGroup = "com.example.NOTI_GROUP";
Step 02. 발송할 Notification에 setGroup으로 지정해줍니다.
Notification notiBuild = new NotificationCompat.Builder(this, channelId)
.setSmallIcon(R.drawable.ic_stat_name)
.setSound(defaultSoundUri)
.setSubText("1번째 줄입니다.") // 1번라인
.setContentTitle("2번째 줄입니다.") // 2번라인 제목
.setContentText("3번째 줄입니다.") // 3번라인 내용
.setStyle(new NotificationCompat.InboxStyle()
.addLine("펼친경우 1번줄입니다.")
.addLine("펼친경우 2번줄입니다.")
.setBigContentTitle("펼친경우 타이틀입니다.")
)
.setGroup(notiGroup);
Step 03. Summary Notification을 생성해줍니다.
NotificationCompat.Builder summary = new NotificationCompat.Builder(this, channelId)
.setContentTitle(title)
.setSmallIcon(R.drawable.ic_stat_name)
.setGroup(notiGroup)
.setGroupSummary(true); // 해당 알림이 Summary라는 설정
Step 04. 알림 시퀀스아이디를 불러와 개별 알람이 되도록 작업해줍니다.
- 이 부분에서는 SharedPreference가 사용되었는데, 이 부분에 대해서는 아래의 게시글에서 확인 가능합니다.
2020/07/22 - [개발 창고/안드로이드 개발] - [Android] SharedPreference 활용하기
SharedPreferences pref = getSharedPreferences(Constants.KEY_PREF, MODE_PRIVATE);
// 마지막으로 저장된 시퀀스 번호를 가져옴
int notiSeq = pref.getInt(Constants.KEY_NOTI_SEQ, 0);
// 다음 발송될 시퀀스를 생성
notiSeq = notiSeq + 1;
Step 05. API 26(오레오) 버전 이후부터는 Notification에 Channel이 지정되어야 합니다.
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
NotificationChannel channel = new NotificationChannel(channelId, channelName, NotificationManager.IMPORTANCE_DEFAULT);
notificationManager.createNotificationChannel(channel);
notification.setChannelId(channelId);
}
Step 06. 알림을 호출합니다.
notificationManager.notify(notiSeq, notification.build());
notificationManager.notify(0, summary.build());
Step 07. 마지막 알림 시퀀스를 저장합니다.
pref.edit().putInt(Constants.KEY_NOTI_SEQ, notiSeq).apply();
순서대로 작성한 경우 해당 알람이 그룹핑되어 발송되게 됩니다.
<전체소스>
// Step 01. 그룹핑 할 그룹명을 지정해줍니다.
String notiGroup = "com.example.NOTI_GROUP";
// Step 02. 발송할 Notification에 setGroup으로 지정해줍니다.
Notification notiBuild = new NotificationCompat.Builder(this, channelId)
.setSmallIcon(R.drawable.ic_stat_name)
.setSound(defaultSoundUri)
.setSubText("1번째 줄입니다.") // 1번라인
.setContentTitle("2번째 줄입니다.") // 2번라인 제목
.setContentText("3번째 줄입니다.") // 3번라인 내용
.setStyle(new NotificationCompat.InboxStyle()
.addLine("펼친경우 1번줄입니다.")
.addLine("펼친경우 2번줄입니다.")
.setBigContentTitle("펼친경우 타이틀입니다.")
)
.setGroup(notiGroup);
// Step 03. Summary Notification을 생성해줍니다.
NotificationCompat.Builder summary = new NotificationCompat.Builder(this, channelId)
.setContentTitle(title)
.setSmallIcon(R.drawable.ic_stat_name)
.setGroup(notiGroup)
.setGroupSummary(true); // 해당 알림이 Summary라는 설정
// Step 04. 알림 시퀀스아이디를 불러와 개별 알람이 되도록 작업해줍니다.
SharedPreferences pref = getSharedPreferences(Constants.KEY_PREF, MODE_PRIVATE);
// 마지막으로 저장된 시퀀스 번호를 가져옴
int notiSeq = pref.getInt(Constants.KEY_NOTI_SEQ, 0);
// 다음 발송될 시퀀스를 생성
notiSeq = notiSeq + 1;
// Step 05. API 26(오레오) 버전 이후부터는 Notification에 Channel이 지정되어야 합니다.
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
NotificationChannel channel = new NotificationChannel(channelId, channelName, NotificationManager.IMPORTANCE_DEFAULT);
notificationManager.createNotificationChannel(channel);
notification.setChannelId(channelId);
}
// Step 06. 알림을 호출합니다.
notificationManager.notify(notiSeq, notification.build());
notificationManager.notify(0, summary.build());
// Step 07. 마지막 알림 시퀀스를 저장합니다.
pref.edit().putInt(Constants.KEY_NOTI_SEQ, notiSeq).apply();
'개발 창고 > Android' 카테고리의 다른 글
[Android] Toolbar에서 로고 위치 오른쪽으로 보내기 (0) | 2020.10.28 |
---|---|
[Android] ImageView 이미지 크기에 View 크기 맞추는 법 (0) | 2020.10.28 |
[Android] net::ERR_CACHE_MISS에 대한 해결방법 (0) | 2020.09.24 |
[Google Play] 2020년 9월 17일자 안내메일 (0) | 2020.09.17 |
[Android] Background에 있는 어플에 firebase 호출 시 onMessageReceived가 호출되지 않는 오류 처리 (0) | 2020.09.16 |